



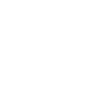
Access our APIs or download our SDK to try our real-time AI models for free.
Download the HANCE VST module player to experience our real-time audio enhancement solutions in any audio editor that supports the VST plugin format. Access our C and Python APIs and their documentation via the links below.
About HANCE
HANCE provides advanced signal-processing techniques developed by experts in machine learning, sound engineering, and audio processing. Our technology enhances audio by removing noise, reverb, and other impairments, offering sound classification and signal recovery—all in real time.
Our APIs make our algorithms accessible on any device or application. With Python and C APIs available—and more platforms coming—developers have the tools to get started. Designed for CPU and memory efficiency, the HANCE APIs provide access to our audio algorithms without sacrificing performance.
Our algorithms combine deep learning and evolutionary computing techniques, enabling systems to learn quickly from auditory data.
We're excited to have you join us. If you have any questions about implementation, please contact us.
Contact HANCE
The HANCE team is excited to have you join us and looks forward to helping you get started. Please feel free to contact us with any questions or concerns about implementation. Our team is available to help as needed.
We thank you for your interest!
HANCE Web API
The HANCE Web API provides developers with a simple, programmatic interface to access HANCE's powerful algorithms and processing capabilities. By making HTTP(S) requests to the HANCE Web API, developers can process audio files, explore the various processing modules HANCE offers, and find the best configuration for their needs.
The HANCE Web API offers developers a taste of the impressive results generated by the HANCE Audio Engine. However, it should be noted that this API does not demonstrate realtime processing. This is because files must be uploaded to the HANCE servers, processed, and downloaded to the user's browser.
We recommend reviewing our C++ and Python API documentation or contacting us directly if you are interested in exploring realtime audio processing or training custom models.
This document provides a comprehensive guide to the HANCE Web API, with endpoints, parameters, options, and JavaScript (ES6) code examples. It is designed to make learning the HANCE Web API quick, easy and fun.
Get Upload URL
To start uploading files from your code, request an upload ID and URL from the server. These will securely handle the file and ensure a successful upload.
https://server.hance.ai/api/get_upload_url.
Get Upload URL: JavaScript Code Example
// First, we store the address from "Important endpoint A" as a constant: const URL_ID_ENDPOINT= " https://server.hance.ai/api/get_upload_url " // Using ES6 syntax, this one-liner downloads and converts our data from JSON // to native JavaScript objects. const getData =async ()=> await (await fetch(URL_ID_ENDPOINT)).json()getData (). then (({ upload_url , upload_id }) => { // Once the data is available, the .then() method will be called, // providing access to the upload_url and upload_id values. Here we would // typically place an upload function, passing in the now available data. // Do something here... // We are also returning the values so that they can be used in the next // steps. return { upload_url, upload_id} } ). catch (console . error )
Get upload URL: query parameters
Field | Type | Description |
---|---|---|
origin | String | Use the origin parameter with your website URL to handle potential CORS issues during web browser file uploads. |
Get upload URL: setting the origin
// Alternative 1: Getting the origin from the current browser URL. let origin= window. location. origin// Alternative 2: Manually setting the origin. let origin= " https://example.com " // Add the origin defined in alternative 1 or 2 as a query parameter to the // endpoint, using the encodeURI method for compatibility when used as a // query parameter. const URL_ID_ENDPOINT= ` https://server.hance.ai/api/get_upload_url?origin= ${ encodeURI ( origin) }`
Get upload URL: return values
Field | Type | Description |
---|---|---|
upload_url | String | The URL used for uploading the file to be processed by the API. |
upload_id | String | A unique identifier that allows the API to identify and process the file. |
Get models
The get_models endpoint provides a list of models you can select to process uploaded audio files. Each model addresses a certain audio-related problem, such as noise reduction, reverb attenuation, signal recovery and so forth.
https://server.hance.ai/api/get_models
Get models: example of returned JSON data
[
{
" model_description": " De-noise",
" model_id": " speech-denoise"
},
{
model_description": " De-reverb",
" model_id": " speech-dereverb"
}
]
Get models: retrieving and selecting a mode
// First, we store the address from Important endpoint B as a constant: const MODELS_ENDPOINT= " https://server.hance.ai/api/get_models " const getModelsData = async () => await (await fetch (MODELS_ENDPOINT)). json ()getModelsData (). then (( models ) => { // The array of objects is now available to the getModelData function // and ready to be utilized. // We can do something with the models data here. To check if we have // received the data, we log it to the console. console . dir (models)// We also return the models data so the values can be used in the next // .then() call, like in the example below. return models} ). then (( models ) => console . log (models[1 ]. model_id))//real_time_noise_reverb_v1 . catch (console . error<)
Get models: return values
Field | Type | Description |
---|---|---|
models | Array of objects | Each object in the models array contain a model_description and a model_id key. |
Processing audio files
Once you have obtained the upload URL and unique ID, as explained in the Get upload URL section, and selected the appropriate model, we can upload audio files to HANCE's servers. To do this, make a POST request to the generated upload URL and include some of the query parameters described below with the request.
Processing audio files: query parameters
Field | Type | Description |
---|---|---|
upload_id | string | The upload_id returned from the Get upload URL endpoint. |
model_id | string | The model used for processing. This can be obtained by using the Get models endpoint detailed above. |
file_type | string | The parameter is optional, and it accepts the two values audio_file or audio_sprite. If no value is provided, it defaults to audio_file. |
return_file_url | string | Option to return the file directly and improve speed by eliminating extra transfer. Set the value to true/false with false as the default value. |
output_format | string | An optional output file format for the processed file. Accepted values are wav (default), mp3, and ogg. |
Processing audio files: return values
The HANCE Web API will return a redirect to the enhanced file once it has completed processing. If the redirect argument is not specified or set to false, the API will return a URL pointing to the processed file instead. The request may take a while to complete, depending on how large and complex the file is.
HANCE C API
The C interface for the HANCE Audio Engine provides developers with simple access to HANCE's powerful algorithms and processing capabilities from all languages that offer bindings for standard C compatible libraries. The HANCE Audio Engine is a light-weight and cross-platform library, and it should be very easy to integrate it into your application. The library can load pre-trained AI models and use these for audio processing to perform various tasks such as noise reduction and de-reverberation.
Getting Started
The HANCE API is designed to be as simple as possible. The ProcessFile example (see the Examples folder in the API) illustrates how to create a HANCE processor and process audio with it. CMake 3.0 or later is required to build the example. To build ProcessFile, open the Terminal (on Mac and Linux) or the Command Line Prompt (on Windows) and locate the Examples/ProcessFile subfolder in the HANCE API. Please type ./Build.sh on Mac or Linux, or Build.bat on Windows.
To use the HANCE API, we first need to make sure the "Include" in the HANCE API is added to the header search path and include the HanceEngine header file:
Include the HANCE Engine Header File
#include " HanceEngine.h "
Now we can create an instance of a HANCE processor by specifying a path to a HANCE model file along with the number of channels and sample rate to use:
Creating a HANCE Processor Instance
// Create a HANCE processor that loads the pre-trained model from file processorHandle= hanceCreateProcessor ( modelFilePath, numOfChannels, sampleRate ); if ( processorHandle== nullptr ) handleError (" Unable to create the HANCE audio processor. ");
The returned processor handle will be nullptr if the processor couldn't be created, e.g., because the model file path is invalid.
Now, we can add audio in floating point format to the HANCE processor. The HANCE API supports audio stored either as channel interleaved audio (hanceAddAudioInterleaved and hanceGetAudioInterleaved) or as separate channel vectors (hanceAddAudio and hanceGetAudio). We demonstrate how to add audio from a std::vector containing channel interleaved float values below:
Adding Channel Interleaved Audio to a HANCE Processor
// We read PCM audio from the file in the 32-bit floating point format hanceAddAudioInterleaved ( processorHandle, audioBuffer. data(), audioBuffer. size() / numOfChannels);
The processing introduces latency, so we need to query how many samples (if any) that are available before we can pick up the processed audio using hanceGetAudioInterleaved:
Getting Processed Audio from a HANCE Processor
int numOfPendingSamples= hanceGetNumOfPendingSamples ( processorHandle ); vector< float > processedBuffer ( numOfChannels* numOfPendingSamples ); if (! hanceGetAudioInterleaved ( processorHandle, processedBuffer. data() numOfPendingSamples)) { handleError(" Unable to get audio from the HANCE audio processor. "); }
You can add silent audio (all values set to zero) to get the processing tail caused by the model's latency. When you have completed the processing of the audio stream, please make sure to delete the HANCE processor to free its memory:
Deleting the HANCE Processor and Free Memory
hanceDeleteProcessor ( processorHandle);
Performance Considerations
The HANCE Audio Engine is a light-weight and cross-platform library, and it uses either of the following libraries for vector arithmetic if available:
Intel Performance Primitives
Apple vDSP
Datatypes
HanceProcessorHandle
typedef void * HanceProcessorHandle;
The processor handle refers to an audio processor that can process PCM audio in 32-bit floating point using a specified inference model. The handle created using hanceCreateProcessor.
HanceProcessorInfo
struct HanceProcessorInfo{ double sampleRate; int32_t numOfModelChannels; int32_t latencyInSamples; };
Return Type | Member name | Description |
---|---|---|
double | sampleRate | Sample rate used when model was trained. The processor will automatically convert sample rates to match the model. |
int32_t | numOfModelChannels | The true number of channels used in the processing. The processor will automatically convert the channel format to match the model. |
int32_t | latencyInSamples | The maximum latency of the model in samples. |
Functions
hanceAddAudio
void hanceAddAudio ( HanceProcessorHandleprocessorHandle , const float ** pcmChannels, int32_t numOfSamples )
Adds floating point PCM encoded audio from separate channels to the HANCE audio processor.
Parameters | Description |
---|---|
processorHandle | Handle to the audio processor. |
pcmChannels | Pointer to an array of channel data pointers, each pointing to sampled PCM values as 32-bit floating point. |
numOfSamples | The number of samples to add |
hanceAddAudioInterleaved
void hanceAddAudioInterleaved ( HanceProcessorHandleprocessorHandle , const float * interleavedPCM , int32_t numOfSamples )
Adds floating point PCM encoded audio from a single channel-interleaved buffer to the HANCE audio processor.
Parameters | Description |
---|---|
processorHandle | Handle to the audio processor. |
interleavedPCM | Pointer to a 32-bit floating point buffer containing channel-interleaved PCM audio (stereo audio will be in the form "Left Sample 1", "Right Sample 1", "Left Sample 2"...). |
numOfSamples | The number of samples to add |
hanceAddLicense
bool hanceAddLicense ( const char * licenseString )
Adds a license key to the HANCE engine to remove audio watermarking on the output.
Parameters | Description |
---|---|
licenseString | A string containing a license received from HANCE. |
Returns | True, if the license check succeeded, otherwise false. |
hanceCreateProcessor
HanceProcessorHandlehanceCreateProcessor ( const char * modelFilepath , int32_t numOfChannels , double sampleRate )
Creates an audio processor, loads a model file and returns a handle to the processor instance if successful.
Parameters | Description |
---|---|
modelFilepath | Pointer to a zero terminated string containing the file path of the model file to load. |
numOfChannels | The number of channels in the audio to process. |
sampleRate | The sample rate of the audio to process. |
Returns | A valid processor handle on success, otherwise nullptr. |
hanceCreateStemSeparator
hanceCreateStemSeparator () HanceProcessorHandlehanceCreateStemSeparator ( int32_t numOfModels , const char ** modelFilepaths , int32_t numOfChannels , double sampleRate )
Creates an audio processor, loads a model file and returns a handle to the processor instance if successful.
Parameters | Description |
---|---|
numOfModels | The number of model files to load |
modelFilepaths | Pointer to an array of pointers to zero terminated strings containing the file paths of the model files to load. |
numOfChannels | The number of channels in the audio to process. |
sampleRate | The sample rate of the audio to process. |
Returns | A valid processor handle on success, otherwise nullptr. |
hanceDeleteProcessor
void hanceDeleteProcessor (HanceProcessorHandle processorHandle)
Deletes a processor instance.
Parameters | Description |
---|---|
processorHandle | Handle to the processor to delete. |
hanceGetAudio
bool hanceGetAudio ( HanceProcessorHandle processorHandle , float * const * pcmChannels , int32_t numOfSamples )
Gets floating point PCM encoded audio in separate channels after processing. The number of requested samples must be less or equal to the number of available samples as returned by /ref hanceGetNumOfPendingSamples.
Parameters | Description |
---|---|
processorHandle | Handle to the audio processor. |
pcmChannels | Pointer to an array of channel data pointers, each receiving sampled PCM values as 32 bit floating point. |
numOfSamples | The number of samples to retrieve |
hanceGetAudioInterleaved
bool hanceGetAudioInterleaved ( HanceProcessorHandleprocessorHandle , float * interleavedPCM , int32_t numOfSamples )
Gets floating point PCM encoded audio in a single channel-interleaved buffer after processing. The number of requested samples must be less or equal to the number of available samples as returned by /ref hanceGetNumOfPendingSamples.
Parameters | Description |
---|---|
processorHandle | Handle to the audio processor. |
interleavedPCM | Pointer to an array of channel data pointers, each receiving sampled PCM values as 32 bit floating point. |
numOfSamples | The number of samples to retrieve |
hanceGetNumOfPendingSamples
int32_t hanceGetNumOfPendingSamples ( HanceProcessorHandleprocessorHandle )
Returns the number of samples that are ready after model inference. If the end of the stream has been reached, endOfStream can can be set to true to retrieve the number of remaining samples in the processing queue.
Parameters | Description |
---|---|
processorHandle | Handle to the audio processor. |
Returns | Number of completed samples. |
hanceGetParameterValue
void hanceGetParameterValue ( HanceProcessorHandleprocessorHandle , int32_t parameterIndex )
Returns a parameter value.
Parameters | Description |
---|---|
processorHandle | Handle to the audio processor. |
parameterIndex | Index of the parameter to return. The available parameters are defines with HANCE_PARAM as pref |
hanceGetProcessorInfo
void hanceGetProcessorInfo ( HanceProcessorHandle processorHandle , HanceProcessorInfo* processorInfo )
Parameters | Description |
---|---|
processorHandle | Handle to the audio processor. |
processorInfo | Pointer to a HanceProcessorInfo struct that will receive the model information. |
hanceResetProcessorState
void hanceResetProcessorState ( HanceProcessorHandleprocessorHandle )
Resets the processor state and clears all delay lines.
Parameters | Description |
---|---|
processorHandle | Handle to the audio processor. |
hanceSetParameterValue
void hanceSetParameterValue ( HanceProcessorHandleprocessorHandle , int32_t parameterIndex , float parameterValue )
Sets a parameter value.
Parameters | Description |
---|---|
processorHandle | Handle to the audio processor. |
parameterIndex | Index of the parameter to return. The available parameters are defines with HANCE_PARAM as prefix.. |
parameterValue | The new parameter value. |
HANCE Python API
HANCE is a powerful audio enhancement engine that can drastically improve the quality of audio signals in your Python projects. This document explains how to install and use the HANCE Python wrapper.
Installation
Installing the HANCE Python wrapper is easy:
Use pip, the standard Python package installer.
Run the command in the example below from the terminal on macOS or the command line on Windows.
Install HANCE with Pip
python - m pip install hance
How to use
To start uploading files from your code, request an upload ID and URL from the server. These will securely handle the file and ensure a successful upload.
import hance
models = hance. list_models()
print( models)
Basic audio processing
To process an audio file using HANCE, use the process_file function, as shown below.
File processing
import hance models= hance . list_models() hance. process_file( models[ 0 ], input_file_path, output_file_path)
The HANCE engine works without a license but it will leave a watermark on the sound. You can obtain a license for the HANCE engine here. This is an example of processing a file using a license.
Stem Separation
For advanced audio processing, HANCE provides stem separation features. This allows you to isolate and manipulate individual components of an audio track, such as vocals, instruments, etc.
Using StemSeparator for Advanced Stem Separation
The StemSeparator class enables more complex stem separation tasks, using multiple models for different stems. Here’s how you can use it:
import hanceimport soundfileas sfimport numpyas npimport osdef separate_stems(input_file_path):# Separates the stems from an input audio file using selected models with the StemSeparator class. (" Stem separation using Hance engine with StemSeparator class. ") models= [' vocals_separation. hance', ' drums_separation. hance', ' piano_separation.hance', 'bass_separation.hance'] print(" Available models for separation: ") for i, modelin enumerate ( models ): ( f "{ i+1 }. { model }") selected_models= input(" Select models to use by entering their numbers separated by commas (e.g., 1,3): ") selected_models_indices= [ int ( index ) - 1for indexin selected_models . split (',')] model_paths= [ models[ index] for indexin selected_models_indices] input_audio, sr= isf . read( input_file_path, dtype=' float32 ') if input_audio == 1:. ndim# Mono to Stereo if needed input_audio =np sample_rate. tile( input_audio[:, np. newaxis], ( 1 , 2 )) = sr num_of_channels= input_audio engine =. ndimhance stem_separator. HanceEngine() = engine separated_stems. StemSeparator( engine. hance_engine, model_paths, num_of_channels, sample_rate) = stem_separator path, fn =. process( input_audio) tos.path . split( input_file_path) for i, model_pathin enumerate ( model_paths ): stem_name= model_path . split(' _ ')[ 0 ] output_file_path =os . path. join( path, f "{fn.split('.')[0]}_{stem_name}_separated.wav") sf . write( output_file_path, separated_stems[:, i* num_of_channels:( i+1 )* num_of_channels], sr) ( f "Stem {stem_name} saved to {output_file_path} ") (" Stem separation completed. ") separate_stems( path_to_file)
Using with a license
import hance license_string= " example_license" models= hance . list_models () hance . process_file( models[ 0 ], input_file_path, output_file_path, license_string = license_string )
Realtime processing
In addition to processing audio files, HANCE can also be used on realtime audio streams. The following example demonstrates how to use HANCE with PyAudio to record audio from a microphone, process it in realtime, and output it to headphones. At the very bottom you can find a minimal example of this with only the HANCE related parts.
Complete Python example of realtime processing
# We capture the input of your microphone and process it in real-time. # We then send it to the output device. import threadingimport numpy as npimport hanceimport pyaudio engine= hance. HanceEngine() p= pyaudio. PyAudio() FORMAT= pyaudio. paFloat32 CHANNELS= 1 RATE= 44100 CHUNK= 12 ("\n Record audio from a microphone and process it in realtime with HANCE. ") (" PyAudio will induce some latency with the roundtrip to the soundcard, ") (" but the HANCE engine runs in realtime. \n") # We get a list of available input devices. input_devices= [] for iin range( p. get_device_count()): device_info = p.get_device_info_by_index(i)if device_info["maxInputChannels"] > 0: input_devices.append(device_info)# Print the list of available input devices and ask the user to select one. (" Available input devices: ") for i, devicein enumerate ( input_devices ): ( f "{ i } : { device [' name ']}") input_device_index= int ( input("\n Select an input device by entering its number: ")) input_device_info= input_devices[ input_device_index] # We get a list of available output devices. output_devices= [] for iin range device_info( p. get_device_count()): = p if device_info. get_device_info_by_index( i) [" maxOutputChannels "] > 0 :output_devices . append( device_info) # We print the list of available input devices and ask the user to select one. ("\n >Available output devices: ") (" To prevent feedback, select a headphones output. \n") for i, devicein enumerate ( output_devices): ( f "{ i} : { device[' name']}") = int ( input ("\n Select an output device by entering its number: ")) output_device_info= output_devices[ output_device_index] models= hance . list_models() processor= engine . create_processor( models[ 0 ], CHANNELS, RATE) stop_thread= False processor_active= True def record_and_playback_thread (): stream_record= p. open( format = FORMAT, channels = CHANNELS, rate = RATE, input = True , input_device_index = input_device_info [' index '], frames_per_buffer = CHUNK) stream_play= p . open( format = pyaudio. paFloat32, channels = 1 , rate RATE= , frames_per_buffer = CHUNK,output = True,output_device_index = output_device_info [' index '] ) while not stop_thread: data= stream_record . read( CHUNK, exception_on_overflow =False ) audio_buffer= np . frombuffer( data, dtype = np. float32) if processor_active: audio_buffer =processor t. process( audio_buffer) stream_play. write( audio_buffer. astype( np. float32). tobytes()) # We stop recording. stream_record. stop_stream() stream_record. close() stream_play. stop_stream() stream_play. close() = threading . Thread( target = record_and_playback_thread) t . start() ("\n The microphone and processing is active ") while True: user_input= input (" Enter 'p' to toggle processing on and off or 'q' to quit: ") if user_input . lower() == " p ": # Bypass processing and continue the loop if processor_active: processor_active= False (" The processing is bypassed ") else : processor_active =True (" The processing is active ") elif user_input . lower ()== " q ": # Stop the thread stop_thread= True break t. join
Here is a minimal example of the HANCE-related code for you to look at:
Minimal Python example
import hanceimport numpyas np engine= hance models. HanceEngine() = hance processor. list_models() = engine . create_processor( models[ 0 ], CHANNELS, RATE) audio_buffer= np . frombuffer( PCM_AUDIO_DATA, dtype = np . float32) audio_buffer= processor . process( audio_buffer)
The HANCE Python wrapper is a powerful tool for audio processing and enhancement. With the ability to remove noise, decrease reverb, and be highly CPU and memory-efficient, HANCE stands at the cutting edge of audio enhancement technology.
FAQ
Frequently Asked Questions
-
What exactly does HANCE do for audio in real-time?
HANCE enhances audio in real-time by removing noise and reverb, boosting voices, and separating instruments from mixed music. It's designed for tech companies involved in microphones, audio gear, video conferencing, podcasting, and streaming software, offering years of development savings and tailored integration.
-
How does the HANCE Audio Engine work?
Developed by AI specialists, sound engineers, and audio processing experts, the HANCE Audio Engine uses extensive algorithms to reduce noise, remove reverb, recover signals, and classify sounds effectively in real-time. It's highly trainable, adaptable, and efficient in CPU and memory usage.
-
Can HANCE be integrated into my current product or service?
Absolutely! HANCE is built to be versatile and can be integrated into a wide range of products and services, from hearing aids to supercomputers. Our team works closely with you to ensure smooth and fast integration, tailoring our technology to meet your specific business needs.
-
What makes HANCE different from other audio enhancement solutions?
HANCE stands out due to its hyper-efficient algorithms that ensure minimal CPU and memory footprint while delivering high-quality audio enhancement. Our solutions are highly adaptable and have been refined through years of R&D, making them suitable for a wide range of applications.
-
Who can benefit from using HANCE?
Developers, hardware engineers, and professionals in post-production, industry, and intelligence can all benefit from HANCE. Whether it's for software development, running on hardware with minimal CPU, improving audio quality in media production, or developing real-time audio classification algorithms, HANCE offers versatile solutions.
-
How can I get started with HANCE?
Getting started with HANCE is easy. Contact us through our website to discuss your organization's audio challenges. We'll provide you with the necessary support, resources, and API documentation (available for C++ and Python, with more languages to come) to integrate HANCE into your projects effectively.